Introduction
Imagine building a powerful, scalable web application without the hassle of managing servers or high infrastructure costs.
That is the promise of serverless architecture.
With AWS Lambda and API Gateway, developers can focus on writing code while AWS handles scalability, performance, and security. Whether you are a startup optimizing costs or an enterprise ensuring high availability, serverless computing offers an efficient and cost-effective way to deploy applications.
This guide walks you through building a REST API using AWS Lambda, API Gateway, and Node.js. By the end, you will have a fully functional API without provisioning a single server.
Let’s dive in.
What is Serverless Architecture?
Serverless architecture is a modern cloud computing model that eliminates the need for developers to manage infrastructure. Instead of provisioning and maintaining servers, cloud providers handle all backend operations, allowing developers to focus solely on writing and deploying code. This approach enhances scalability, reduces operational complexity, and optimizes costs.
How serverless works:
- Event-Driven Execution: Developers write code as small, independent functions that trigger in response to specific events.
- Automated Resource Management: Cloud providers dynamically allocate compute resources, ensuring optimal performance and efficiency.
- Pay-Per-Use Pricing: Billing is based on execution time and resource consumption, eliminating fixed infrastructure costs.
Key Components of Serverless Architecture
Serverless architecture eliminates the need for traditional server management, allowing developers to focus on building applications. Here are the essential components that make it work:
1. Function as a Service (FaaS)
FaaS platforms like AWS Lambda and Azure Functions let developers run code in response to events. This eliminates the need to manage servers, ensuring applications scale effortlessly.
2. API Gateway
A serverless API gateway handles routing, authentication, and request processing. Services like AWS API Gateway simplify API creation, making connecting front-end applications with backend logic easy.
3. Event Triggers
Serverless functions execute based on specific events, such as:
- A file upload to AWS S3
- A new entry in DynamoDB
- An HTTP request to an API
This event-driven approach ensures efficiency and real-time responsiveness.
4. Serverless Databases
Managed databases like AWS DynamoDB and Firestore offer scalable, high-performance storage without manual maintenance. They adapt to workload demands automatically.
5. Authentication & Security
Identity and access control services, such as AWS IAM and Auth0, manage user authentication and permissions, ensuring secure interactions within the application.
Businesses can build scalable, cost-efficient, and agile applications by leveraging serverless architecture. With reduced infrastructure overhead, developers can focus on innovation rather than server maintenance.
Understanding AWS Services for Serverless Development
To build a scalable and secure serverless REST API, key AWS services include:
- AWS Lambda: Runs code in response to events without managing servers. It automatically scales and charges only for execution time. Ideal for backend logic in REST APIs.
- Amazon API Gateway: Manages and routes HTTP requests to Lambda. It handles authorization, access control, and monitoring for seamless API management.
- AWS DynamoDB (Optional): A fully managed NoSQL database that integrates with Lambda and API Gateway. It scales automatically and requires no maintenance.
- AWS IAM: Controls access to AWS services, ensuring only authorized users and services interact with your API, Lambda, or database.
You can build a serverless REST API that scales efficiently, remains secure, and simplifies operations using these services.
Setting Up AWS for Serverless Development
To build serverless applications on AWS, you need to configure your AWS environment correctly. Follow these steps to get started.
1. Creating an AWS Account
Before using AWS services, you must create an account:
- Go to AWS Sign-Up and click on Create an AWS Account.
- Provide your email address, set up an account name, and choose a secure password.
- Enter your billing details and select an AWS support plan (you can start with the Free Tier).
- Verify your identity using a phone number.
- Once verified, sign in to the AWS Management Console.
2. Setting Up IAM Roles and Policies
AWS Identity and Access Management (IAM) controls permissions for accessing AWS services. To allow AWS Lambda to execute functions securely, create an IAM role with the required permissions.
Steps to Create an IAM Role for Lambda:
- Open the AWS Management Console and navigate to IAM (Identity and Access Management).
- In the IAM dashboard, go to Roles and click Create role.
- Select AWS Service as the trusted entity type and choose Lambda as the service.
- Click Next to attach permissions. Search for AWSLambdaBasicExecutionRole and select it.
- Click Next: Tags (optional) and then Next: Review.
- Provide a meaningful role name (e.g., LambdaExecutionRole) and click Create role.
Your Lambda functions can now assume this IAM role to interact with AWS resources.
3. Installing AWS CLI and AWS SDK
To manage AWS services from your local machine, install:
- AWS CLI (Command Line Interface) for executing AWS commands.
- AWS SDK (Software Development Kit) for integrating AWS with your applications.
Installing AWS CLI
AWS CLI allows you to interact with AWS services from your terminal or command prompt.
1. Download the AWS CLI installer:
- Windows: AWS CLI for Windows
- Mac: Install using Homebrew:

- Linux: Install using the package manager:
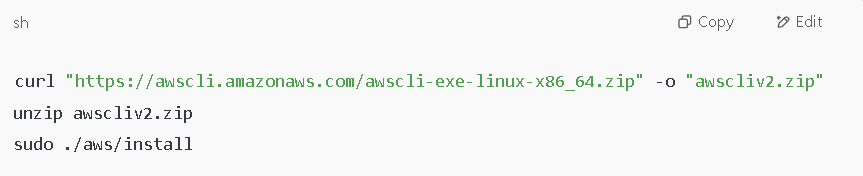
2. Verify the installation:

Configuring AWS CLI
Run the following command and enter your AWS credentials:

You will be prompted to enter:
- AWS Access Key ID
- AWS Secret Access Key
- Default region (e.g., us-east-1)
- Default output format (choose json, table, or text)
Installing AWS SDK for Node.js
If you are developing a serverless application in Node.js, install the AWS SDK:

This SDK allows your application to interact with AWS services like S3, Lambda, and DynamoDB.
Now, your AWS environment is ready for serverless development.
Building a REST API with AWS Lambda and API Gateway
AWS Lambda and API Gateway allow you to create serverless REST APIs without managing servers.
This guide walks you through building and deploying a simple REST API using Node.js.
Step 1: Creating a Lambda Function with Node.js
1. Open AWS Lambda Console
- Sign in to the AWS Management Console.
- Navigate to AWS Lambda and click Create function.
2. Define a New Lambda Function
- Choose Author from scratch.
- Set a function name (e.g., HelloWorldFunction).
- Select Node.js as the runtime.
- Choose an existing IAM role with Lambda execution permissions (or create a new one).
- Click Create function.
3. Write a Simple Lambda Function
Once the function is created, replace the default code with the following:
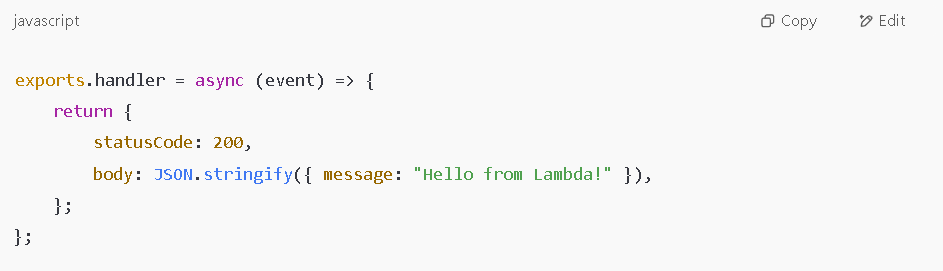
This function responds with a JSON object containing a greeting message.
Step 2: Deploying the Lambda Function
- Click Deploy to save and apply the function changes.
- Copy the Lambda function ARN (Amazon Resource Name) for later use in API Gateway.
Step 3: Configuring API Gateway
API Gateway acts as an entry point for HTTP requests to invoke Lambda functions.
1. Create a New API
- Open the API Gateway console.
- Click Create API and select HTTP API or REST API.
- Choose New API and enter an API name (e.g., HelloWorldAPI).
- Click Create API.
2. Define an API Resource and Method
- In the API Gateway dashboard, select Resources.
- Click Create Resource and enter a resource name (hello).
- Under the newly created /hello resource, click Create Method.
- Select GET and click ✔️ (checkmark) to proceed.
Step 4: Connecting API Gateway to Lambda
1. Set Up Lambda Integration
- For Integration type, choose Lambda Function.
- Enter the name of your Lambda function (e.g., HelloWorldFunction).
- Click Save and confirm when prompted to permit API Gateway to invoke the Lambda function.
2. Deploy the API
- Click Deploy API.
- Create a new stage (e.g., dev) and click Deploy.
- Copy the Invoke URL provided by API Gateway.
Step 5: Testing the REST API
You can test your API using a web browser or API tools like Postman.
1. Send a GET request to:

If everything is set up correctly, you should receive the following response:

Now, you have successfully built and deployed a serverless REST API using AWS Lambda and API Gateway.
Debugging with AWS CloudWatch
AWS CloudWatch logs help track API requests and errors.
- Go to AWS Lambda > Monitoring > View logs in CloudWatch.
- Check logs for errors and performance data.
Deploying and Securing the API
Deploy API Gateway with Stages
Stages help manage different environments:
- dev For testing.
- staging For pre-release testing.
- prod For live users.
To deploy:
- In API Gateway, go to Deployments.
- Click Deploy API, select a stage, and deploy.
Secure API with Authentication
- AWS Cognito: Adds user authentication.
- API Keys: Restricts access.
To enable API keys:
- In API Gateway, go to your API method (e.g., GET /hello).
- Under Method Request, enable API Key Required.
- Create an API key under API Keys.
Enable CORS
To allow cross-origin requests:
- In API Gateway, go to your API resource.
- Click Actions > Enable CORS and configure allowed methods.
- Deploy the API.
Optimizing and Scaling
1. Reduce Cold Starts
- Use Provisioned Concurrency to keep Lambda warm.
- Optimize function size and dependencies.
2. Enable API Caching
- In API Gateway, go to Stages.
- Select your stage and enable Caching.
- Set a TTL (time-to-live) to reduce redundant requests.
3. Monitor and Improve Performance
- Use AWS X-Ray to analyze API response times.
- Track execution logs with CloudWatch Metrics.
- Optimize Lambda code for faster execution.
Following these steps ensures your API is fast, secure, and scalable.
Conclusion
Serverless architecture simplifies development by removing infrastructure management, cutting costs, and boosting scalability. Using AWS Lambda, API Gateway, and Node.js, you can build efficient, secure REST APIs with minimal maintenance. As businesses embrace cloud-native solutions, serverless technology ensures flexibility and growth.
Need expert help in building high-performance serverless applications? MeisterIT Systems specializes in AWS, Node.js, and cloud computing.
Contact us today to streamline your development and accelerate business growth!